Learning object-oriented programming (OOP) can be made easier with a structured approach and plenty of practice. This guide will help you step by step:
1. Understand the Basics of OOP:
– Learn about the core concepts of OOP: classes, objects, inheritance, encapsulation, and polymorphism. These are the building blocks of OOP.
– Understand how objects interact with each other through methods and properties.
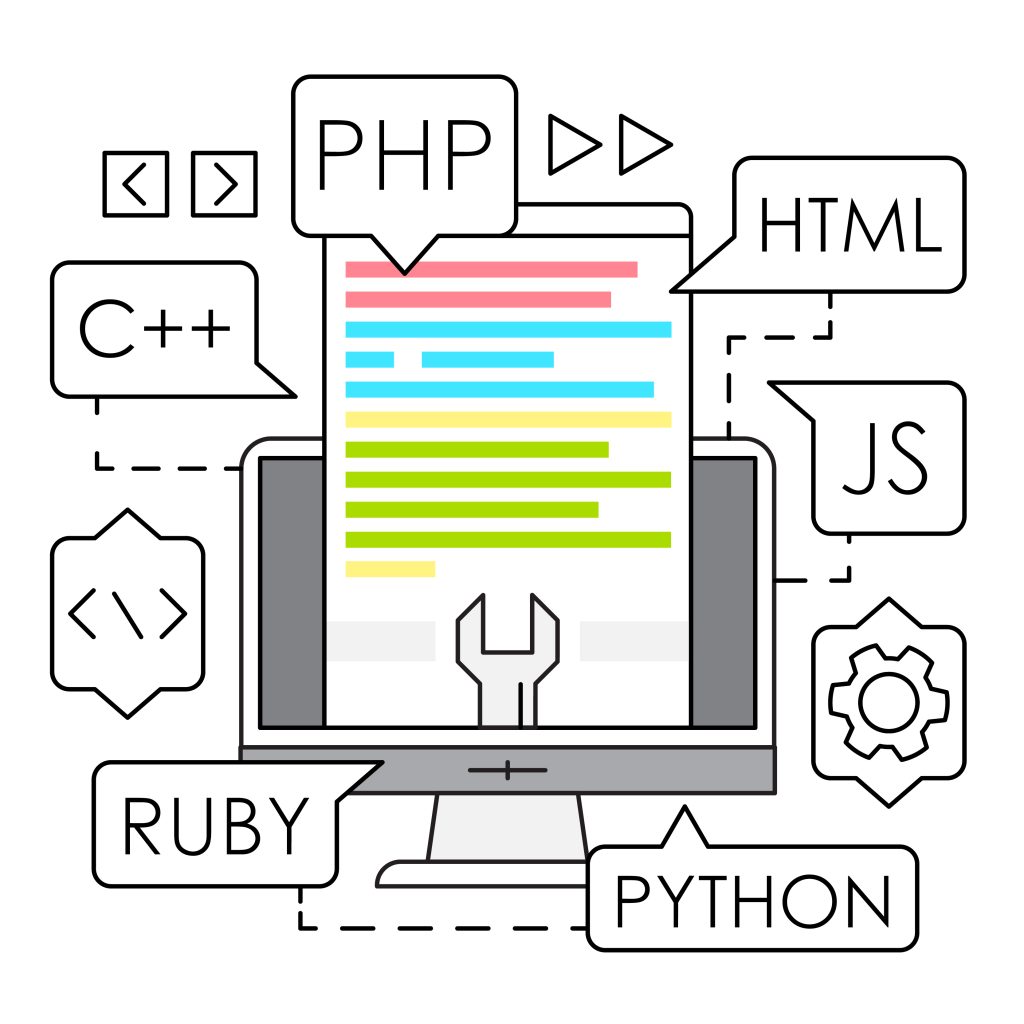
2. Choose a Programming Language:
– Pick a programming language that supports OOP well. Popular choices include Python, Java, C++, and C#.
– Python is often recommended for beginners due to its simple syntax and readability.
3. Start with Simple Examples:
– Begin with simple programs to create classes and objects. For example, create a class representing a person with attributes like name and age.
– Practice creating objects from these classes and calling their methods.
4. Learn about Classes and Objects:
– Understand the concept of classes as blueprints for creating objects. Objects are instances of classes.
– Learn how to define classes, add attributes (properties), and methods (functions).
5. Explore Inheritance and Polymorphism:
– Understand inheritance, where a class can inherit attributes and methods from another class. This promotes code reuse.
– Learn about polymorphism, where different classes can be treated as instances of the same class, enabling flexibility in code design.
6. Practice Designing Object-Oriented Systems:
– Work on small projects or exercises that require you to design systems using OOP principles. This could be a simple game, a student management system, etc.
– Break down problems into smaller, manageable parts and design classes to represent these parts.
7. Read and Write Code Regularly:
– Read code written by others to understand different approaches to solving problems using OOP.
– There is no option without practice coding regularly to improve your skills.
8. Utilize Online Resources and Tutorials:
– There are numerous tutorials, articles, and videos available online that cover OOP concepts in various programming languages.
– Websites like Codecademy, Udemy, and Coursera offer courses specifically focused on teaching OOP.
9. Join Coding Communities:
– Engage with coding communities and forums where you can ask questions, seek help, and participate in discussions related to OOP.
– Collaborate with others on projects to gain practical experience and learn from more experienced developers.
10. Stay Patient and Persistent:
– OOP can be challenging at first, but with persistence and practice, you’ll gradually become more comfortable with its concepts and principles.
– Don’t get discouraged by initial difficulties. Keep practicing every day. Don’t hesitate seeking help when you stuck.
Remember, learning OOP is a journey, and it’s okay to take your time to fully grasp its concepts. Start from small to build a solid and strong foundation. Day by day expand your knowledge and skills.
Pingback: Object Oriented Programming | Sanjoy Dey Reju